If you’re looking to build a website using Gatsby and Contentful you’ve come to the right place.
In this guide you’ll mainly be focusing on the sourcing of data and learning how gatsby-source-contentful works rather than how to make a pretty looking website.
The finished Quick Start site can be seen here:
Demo: https://contentfulquickstart.gatsbyjs.io
Repo: https://github.com/PaulieScanlon/contentful-quick-start
…and yes, it doesn’t look pretty at all, but that’s fine, my approach is to always start with the data! always, always start with the data!
Some prerequisites if you’d like to follow along.
- Contentful
- You’ll need a Contentful account
- You’ll need the space id
- You’ll need the access token
- You’ll need some content in Contentful to query (i’ll show you how)
- Gatsby
- You’ll need a basic Gatsby site, here’s an absolute bare-bones repo you can clone if you like: gatsby-demo-minimal
Contentful
Once you have a Contentful account you’ll need to create a “space” you can do this from the main menu — look for a button that says “+ Add space”.
Space
Give your space a “Space name” and leave the default “Create an empty space” radio button checked, then click “Proceed to confirmation”.
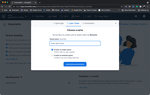
Give your space a “Client name” and “Short project description”.
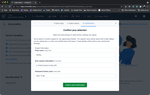
Ignore this screen!
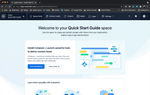
Content Model
Before you get to adding content you’ll first need to define some fields that hold the content.
In Contentful this is called the “Content Model”.
Go to “Content Model” from the top menu, and click the button that says “Add content type”.
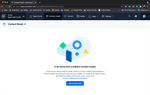
Give your Content Type a “Name”, “Api identifier” and a “Description”, for the purposes of this Quick Start guide make sure the “Name” is “Page” as you’ll be referring to this later.
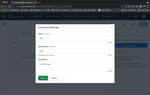
When you’re done click “Create”.
Next, you’ll need to add some fields by clicking on the button that says “+ Add field”.
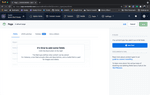
You can add whatever fields you like but for this Quick Start guide I’ve added x4 fields, the only one that really matters if you’re following along is the url
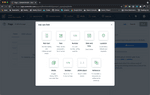
The fields to add are as follows:
- url
- This is of type Text – with a sub selection of “Short text”
- On the “Validation” tab make sure this is set to “Required Field”
- title
- This is of type Text – with a sub selection of “Short text”
- description
- This is of type Text – with a sub selection of “Long text”
- image
This is of type Media
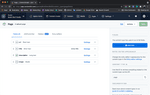
Content
Now you have a “Content Model” you can add content using this model.
Go to “Content” from the top menu, and click the button that says “Add Content Type” then click the button that says “Add Page”.
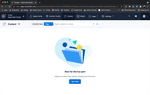
You should now see that the “Page” contains fields that you defined in the “Content Model”.
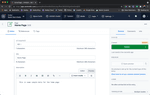
Fill them out and click “Publish”.
While you’re there it’s also worth creating an About Page with the url value set to “about”.That’s the end of adding content to Contentful, next you can query this data using gatsby-source-contentful.
Gatsby
Clone the gatsby-demo-minimal repo and install the dependencies, maybe spin it up just once to make sure there’s no errors but more importantly make sure you can see the GraphiQL Explorer (pronounced graphical) on this url http://localhost:8000/___graphql.
You can use the following allSitePage
query just to confirm everything is working correctly.
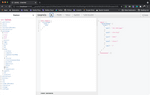
What you’re looking at here is a graphical representation of Gatsby’s data layer. This is where everything happens. GraphiQL is interactive too, you can click around in the Explorer on the left to create different types of GraphQL queries. To see the result of the query click the play icon button.
The above query returns paths to pages found in your Gatsby site. The image shows there are pages for the following:
- “/dev-404-page/”
- “/404.html”
- “/404/”
- “/about/”
- “/”
You won’t actually need the “/” or “/about” pages since pages will be sourced from Contentful so go ahead and delete both index.js
and about.js
from the /src/pages
directory.
In the next step you’ll be adding gatsby-source-contentful and then you’ll come back to GraphiQL to query the new data that gets sourced by the gatsby-source-contentful plugin.
gatsby-source-contentful
There’s a fair amount of documentation for this plugin already, here’s two good resources for you to read over.
Both of those resources cover how to install and configure the plugin so I won’t repeat those steps in this guide.
You will however probably want to have a glance at this page from the docs which covers how to use Environment Variables.
As mentioned in the Contentful plugin docs the two variables you’ll need are the spaceId
and the accessToken
, both of these can be found in Contentful by navigating to Settings > API Keys
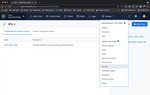
To use your API keys create a .env.development
file at the root of your project and add the following and replace with 123 and abc with the keys from your Contentful API keys settings.
Then update your gatsby-config.js
with the names of your environment variables. For reference here’s the gatsby-config.js from my Quick Start repo
With the Gatsby development server running it’s now time to pop back over to GraphiQL.
As you did before with the allSitePage
query you’ll now use the allContentfulPage
query in GraphiQL to query all pages sourced from Contentful — there’s one other small difference here. I named the field url
in the content model because path
is reserved by Gatsby.
Which should result in something similar to the below.
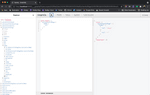
There’s not an awful lot going on here but it does at least show that you are able to query the pages you created in Contentful earlier and the url field returns the correct value for each.
Pages
To create Gatsby pages from the page data sourced from Contentful you’ll need to understand two concepts
Collection Routes
Collection routes are effectively file names escaped by curly brackets that can be associated with a field name in the Gatsby data layer.
The field you’ll be using for creating an actual Gatsby page from Contentful page data is called contentfulPage.url
...Page
comes from the name you gave to the content type in Contentful, (Gatsby prefixes this withcontentful
)-
url
is the required field you added earlier to the Content Model
To use collection routes, create a new file in /src/pages
and call it {contentfulPage.url}.js
, and for reference here’s the {contentfulPage.url}.js from my Quick Start repo
Now, the next bit is a bit tricky and for the purposes of completeness my explanation is a two part process so bear with me.
As you’ve seen already you’ve been using allContentfulPage
to query all pages, but to query data for a one page you’ll need to use the singular version, this query is called contentfulPage
.
However, when using the singular query GraphQL must be passed a value, e.g an id
to use as a lookup key to locate the correct data object in Gatsby’s data layer.
Step 1 All Page Query
This is a temporary measure to help explain step two.
Using the below query you’ll be able to see all the pages returned by Contentful, notice now you’re only querying for the field name id
— you’ll need this in a moment
Which should result in something similar to the below.
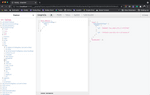
Make a note of one of the id’s, perhaps copy it and save it somewhere before moving to Step 2.
Step 2 Page Query (Singular)
Using GraphiQL again, create a new query and call it pageQuery
, this query will pass an id
value that you can define in GraphiQL’s QUERY VARIABLES, and yep you guessed it, the id
is the one you copied and saved earlier.
The reason you need an id
passed as a parameter is because page queries are unique.
Using the id
in this way acts a bit like a filter and ensures the correct data gets passed to each page.
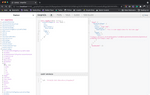
You can see from the above image that by passing an id
into the pageQuery
will result in only the data relevant for that page being returned.
The Page
To turn this query into something more useful you’ll add the above pageQuery
to the {contentfulPage.url}.js
collection route file,
The below code snippet consists of the following:
- A React Function Component that is set to
export default
- A
data
const that contains the GraphQLpageQuery
- A de-structured prop called
data
- An HTML
<pre>
element that renders thedata
If you now visit http://localhost:8000/ you should be looking at something as delightful as the below, oh and if you also created an about page you’ll be able to see that on http://localhost:8000/about.
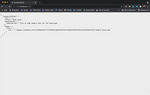
As promised right at the beginning this page isn’t very pretty, but there are a few things you can do to make this page look ever so slightly nicer.
To tidy this page up a bit have a look at the diff below.
Et voilà! This completes my Quick Start guide. I hope that by diving a little deeper on your first pass with Contentful and Gatsby you’ll have a better understanding of how these two technologies can be used together, but if you do have any questions please feel free to find me on Twitter: @PaulieScanlon.
Ttfn
Paul 🕺